So for the midterm I decided I wanted to something with finding poorly secured WIFI networks with the portability of the ESP8266.
My original plan was to use a GPS module I had picked up and once I had found a weakly protected network I would send the SSID, Password, and coordinates up to a web page. Then the webpage would be able to display a map of all the networks found with the corresponding SSID and password so if you ever knew you were going to be around a certain area and wanted to access a wifi network you could.
Big problem I encountered is that the GPS module was giving me basically no real readings about the coordinates. I dunno if the board is just faulty or if it isn’t able to pick up signal that well within the city but supposedly the type I picked up was meant for automobiles so I would think it would work a little better than that. (part = ublox GPS6MV2)
SO I scrapped the GPS part of the project for now and continued to work with the finding weak passwords part of the device. The difficulty here is that there are a limited number of passwords I would be able to test for each WIFI network for two reasons: first, it sometimes takes up to 5 seconds to connect to the WIFI network from the ESP8266 and therefore we would have to wait until five seconds have passed in order to judge the validity of each password guess, and second, there is very limited memory on the ESP8266. Because of the first problem we know we can’t do a full brute-force which would check every possible letter/number combo and because of the second problem we are limited to the number of password guesses we can store within the memory natively. That being said, I used a list I found of the top 100 most commonly found wifi passwords.
Now the plan is to attempt to crack the password using one of the most common passwords and once I successfully connect to a wifi-network I would send up the SSID and password to the web server. This would of course work theoretically because once connected to the wifi network the ESP8266 would have internet access and could make any GET or POST request it wants.
Here are pictures of the code responsible for the POST request from the microcontroller and the serverbside code which gets the data and stores it:
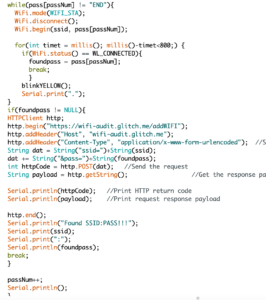
And here is an example of the password cracking in action (it actually works!):
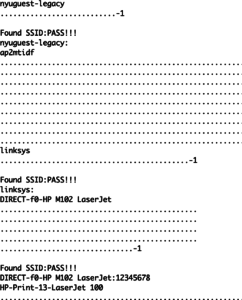
Minor problem thought, haven’t quite figured out how to make the HTTP requests go through. For some reason the client keeps on failing to connect but this should be fixable. Here is a screenshot of a request and response I simulated through Burp Suite to ensure the server is working appropriately:
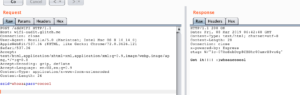
I think this is going to be a really cool project but I need to solidify a couple things first. One main thing is that if it finds a “network” and corresponding password but that network isn’t a true network for internet access (such as a printer network as shown in screenshot) or there is an additional authentication mechanism (as in nyu-guest) then it won’t be able to send that request to the server. What it should do instead is store the found credentials and send them when it finds a network which it can reach the internet through. Or it could drop them because they aren’t necessarily useful for gaining network access but maybe could be useful for something else so I think its best to keep them in consideration for now. Additionally there is the question of if I want to keep pursuing the GPS aspect of the project. I think this is probably the coolest part of it considering without it all that is visible are a list of SSIDs and passwords but we have no idea where they are. This is fine if we just wanna check the ones in our surrounding area but say I wanted to jump on my skateboard and ride around the city finding weak wifi passwords, at the end I would just have a list of SSID/Password combos and not know where to go to find the WIFI networks. There is a arduino library I found which uses surrounding wifi networks and one wifi network which I can connect to in order to determine the coordinates using a google API. I can’t say how reliable this is but it’s a possibility and I would definitely be interested in trying to get it to work with GPS somehow.